It started happening while I was still at college. We students have informally divided into two groups, us that were constantly digging for more knowledge and them that were telling us that we will just be stupid programmers. We wanted to be great at software development, but they said that we will be zombies staring at out monitors for the rest of our lives. They had different future in mind for them, where they would be very smart without any effort, work little and be very good paid for that. My favorite quote from them was "I won't be a programmer, I will be a consultant to other programmers" ... straight from collage. Imagine that! Or "I will stay at the collage and teach others on the advanced software development techniques"... techniques they have never experienced.
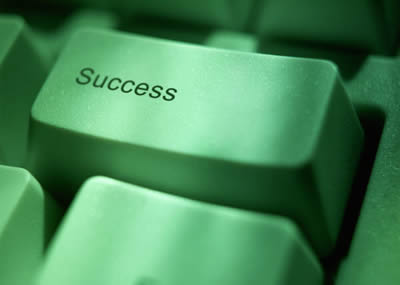
Think of some person you admire for what they have achieved in their profession. Do you think they earned your respect by being talented or with their passion and hard work? Do you think they were stupid to push so hard?
Albert Einstein was committed to his work but I never heard anybody says that he was staring at formulas for the whole day. Nobody complained that he was always lost in his taught or that his clothes were always wrinkled. And what about Jimmy Hendrix, when you think of him, do you think he was a freak locked up in a room all day long with his guitar? What about Michael Jordan, can you even imagine his commitment to basketball? Do you know he was rated as untalented player when he was in high school?
The reality check
The key point that I am trying to make here is that you can't be great at anything if you don't commit to it. There is a myth of talent and intelligence, saying that success only depends on it and that you either have it like gift from the God or not at all. From my personal experience and people around me I believe that talent and intelligence have nothing to do with success. Fortunately, research results confirm my thoughts:
"Research now shows that the lack of natural talent is irrelevant to great success. The secret? Painful and demanding practice and hard work.
...
The critical reality is that we are not hostage to some naturally granted level of talent. We can make ourselves what we will. Strangely, that idea is not popular. People hate abandoning the notion that they would coast to fame and riches if they found their talent. But that view is tragically constraining, because when they hit life's inevitable bumps in the road, they conclude that they just aren't gifted and give up."
And for all of you dreaming of building a startup and getting rich, you should be prepared for hard work because according to Paul Graham, determination is the key factor for startup success.
"We learned quickly that the most important predictor of success is determination. At first we thought it might be intelligence. Everyone likes to believe that's what makes startups succeed. It makes a better story that a company won because its founders were so smart. The PR people and reporters who spread such stories probably believe them themselves. But while it certainly helps to be smart, it's not the deciding factor. There are plenty of people as smart as Bill Gates who achieve nothing.
In most domains, talent is overrated compared to determination—partly because it makes a better story, partly because it gives onlookers an excuse for being lazy, and partly because after a while determination starts to look like talent."
Geoff Colvin describes what deliberate practice is in his book Talent is Overrated:
"Deliberate practice is activity designed specifically to improve performance, often with a teacher’s help; it can be repeated a lot; feedback on results is continuously available; it’s highly demanding mentally, whether the activity is purely intellectual, such as chess or business-related activities, or heavily physical, such as sports; and it isn’t much fun.
….most of us, as adults, are just doing what we’ve done before and hoping to maintain the level of performance that we probably reached long ago. Deliberate practice requires that one identify certain sharply defined elements of performance that need to be improved, and then work intently on them."
If you don't believe it, see Deliberate Practice in Software Development, excellent presentation that takes scientific data to show that in any work (sport, art, engineering…) there is no substitute for hard work and practice.
Einstein advices
So to get back to Einstein, he left us with his 10 universal principles that can be applied to any work where someone wants to achieve excellence. So here are some of the things one of the greatest scientists of all time had to say:
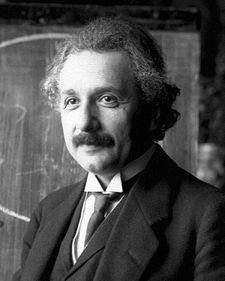
On Determination
“I have no special talent. I am only passionately curious.”
“It's not that I'm so smart; it's just that I stay with problems longer.”
On Value
“Strive not to be a success, but rather to be of value."
On Experience
“Information is not knowledge. The only source of knowledge is experience.”
“A person who never made a mistake never tried anything new.”
On Self-improvement
“Insanity: doing the same thing over and over again and expecting different results.”
“You have to learn the rules of the game. And then you have to play better than anyone else.”
Conclusion
To sum it up, If you want to be great at something (even programming) you need to commit to it. You should Do something and then get better at it.
So the question is do you want to be excellent at your work and what are you doing about it? Are you better than others? Do you have skills that will bring value to your employers/clients? What are you currently trying to learn/improve?
Remember, E=MC2 was not genius, it was hard work.